Hello everyone who came here to read this blogpost. You all came here to know how much JavaScript you should know to getting stated with react JS. Then you had came to right place. So in this post i will explain how much javascript you should know for react js.
If you are a beginners in web development journey, And want to learn react or create a website with React JS. I had explained everything you need to know to start your react js journey. Before you start learning react you should have a basic knowledge of HTML, CSS & JS.
So in this post I will covering all the topic that you should know inside JS before you start learning React JS. If you will cover all this topic which i will explain in this post. Then your react learning journey will become very smooth. This will be very helpful for you and this is the right path to master React JS.
What Should To Know Before Learning React JS
So basically before learning react you should have a basic knowledge of HTML & CSS. Because HTML & CSS is the backbone of all the website you create. When the any web app you will make the HTML & CSS is the main thing which will render on DOM.
But the major thing for getting started with react is JavaScript. And most of the people have a question How much JS you should know?. So this Post is primary focused on how much JS topic which is necessary you should know. Now let’s cover this topic one by one and i will also tell you how much you should learn from this topic. I will start from basic to advance, So this post can clear you all question related to learning of react js
Basics Of JavaScript For React JS
So our first topic is how much basic of JS you should know to getting started with react. Let’s take a how variable works, There are different type of variable in javascript. And at lest you should know all the type of variable which is in JS.
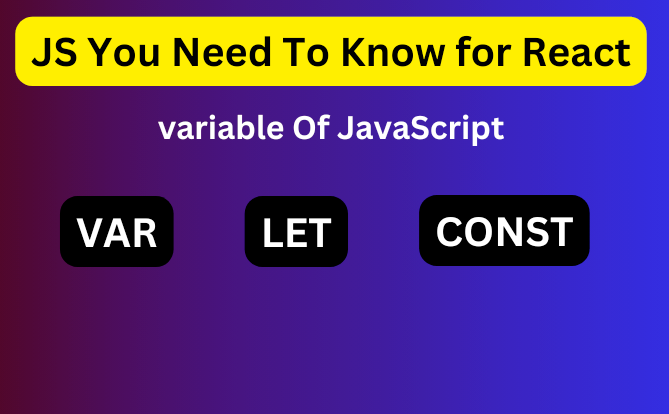
These are the some Variable of JS
- var
- const
- let
These are the all some variable of javascript which you should know about them. Because This is all a basic thing of JS. And this is needed to start your react development journey. So we will also understand each and every variable in detail.
What is Var variable in JS?
JavaScript provides multiple ways to declare variables, with var
being one of the oldest and most traditional methods. Before the introduction of let
and const
in ES6 (2015), var
was the only way to declare variables. While still functional, it comes with certain unique characteristics that modern developers should understand.
we’ll explore the behavior of var
, its quirks, and its limitations, and provide examples to clarify its usage.
function exampleVarScope() {
var x = 10; // Declared within the function
if (true) {
var y = 20; // Still within the function scope, not block scope
}
console.log(x); // Output: 10
console.log(y); // Output: 20 (accessible because of function scope)
}
exampleVarScope();
In this example, y
is accessible outside the if
block because var
variables do not have block scope—they belong to the enclosing function.
While var
was an essential part of JavaScript’s history, its quirks often lead to unexpected behavior. By understanding its characteristics, such as function scope, hoisting, lack of block scope, and re-declaration allowance, you can navigate legacy codebases effectively.
For new projects, prefer using let
and const
to write more predictable and robust JavaScript code. They are modern, safer, and better suited for today’s development practices
What is Const variable in JS?
JavaScript introduced const
in ES6 (2015) as a modern way to declare variables. Unlike var
, const
allows you to create variables with a constant reference, ensuring that the value cannot be reassigned. It has become a preferred choice for variables that remain constant throughout the program.
We’ll explore the behavior, characteristics, and limitations of const
, with examples to clarify its usage
// Example 1: Block Scope
if (true) {
const x = 10; // Scoped to this block
console.log("Inside block:", x); // Output: 10
}
// console.log(x); // Error: x is not defined
// Example 2: No Reassignment
const a = 5;
// a = 10; // Error: Assignment to constant variable
// Example 3: Mutable References for Objects
const obj = { name: "Alice" };
obj.name = "Bob"; // Allowed
console.log("Updated object:", obj); // Output: { name: "Bob" }
// Mutable References for Arrays
const numbers = [1, 2, 3];
numbers.push(4); // Allowed
console.log("Updated array:", numbers); // Output: [1, 2, 3, 4]
// Example 4: Initialization at Declaration
// const b; // Error: Missing initializer in const declaration
// Example 5: No Re-declaration
const c = 100;
// const c = 200; // Error: Identifier 'c' has already been declared
// Example 6: Using const for Functions
const greet = function () {
console.log("Hello!");
};
greet(); // Output: Hello!
// greet = function () { console.log("Hi!"); }; // Error: Assignment to constant variable
// Example 7: Common Use Cases with Arrays and Objects
const fruits = ["apple", "banana"];
fruits.push("cherry"); // Allowed
console.log("Fruits array:", fruits); // Output: ["apple", "banana", "cherry"]
const car = { brand: "Toyota", model: "Corolla" };
car.model = "Camry"; // Allowed
console.log("Car object:", car); // Output: { brand: "Toyota", model: "Camry" }
Explanation of Code
- Block Scope: The variable
x
exists only inside theif
block. Accessing it outside results in an error. - No Reassignment: The value of
a
cannot be reassigned. - Mutable References: You can modify the contents of objects (
obj
) and arrays (numbers
) declared withconst
. - Initialization at Declaration:
const
variables must be initialized when declared. - No Re-declaration: A variable declared with
const
cannot be declared again in the same scope. - Functions: Functions declared with
const
ensure their reference cannot be reassigned. - Practical Usage:
const
is commonly used for arrays and objects, making it clear that their references remain constant while allowing modifications to their contents.
The const
keyword is a powerful addition to JavaScript, providing a way to declare immutable references and ensuring code consistency. With its block-scoping and prevention of reassignment, const
helps you write cleaner, safer, and more reliable code.
By understanding and using const
effectively, you can embrace modern JavaScript practices and avoid common pitfalls associated with mutable variables.
What is let variable in JavaScript ?
When working with JavaScript, one of the most commonly used keywords for declaring variables is let. Introduced in ES6 (ECMAScript 2015), let provides developers with a flexible and safer way to manage variable declarations compared to the older var. Let’s explore what let is, how it works, and why it is so useful, with practical examples.
Unlike var, variables declared with let are block-scoped, meaning they are only accessible within the block, statement, or expression where they are defined. Additionally, variables declared with let can be updated but not redeclared within the same scope
// Example 1: Block Scope
if (true) {
let x = 10; // Scoped to this block
console.log("Inside block:", x); // Output: 10
}
// console.log(x); // Error: x is not defined
// Example 2: Reassignment
let a = 5;
a = 10; // Allowed
console.log("Reassigned value of a:", a); // Output: 10
// Example 3: Mutable References for Objects and Arrays
let obj = { name: "Alice" };
obj.name = "Bob"; // Allowed
console.log("Updated object:", obj); // Output: { name: "Bob" }
let numbers = [1, 2, 3];
numbers.push(4); // Allowed
console.log("Updated array:", numbers); // Output: [1, 2, 3, 4]
// Example 4: No Initialization Required
let b;
console.log("Value of b before initialization:", b); // Output: undefined
b = 20; // Assigning later is allowed
console.log("Value of b after initialization:", b); // Output: 20
// Example 5: No Re-declaration in the Same Scope
let c = 100;
// let c = 200; // Error: Identifier 'c' has already been declared
// Example 6: Block-Scoped Behavior in Loops
for (let i = 0; i < 3; i++) {
console.log("Inside loop:", i); // Output: 0, 1, 2
}
// console.log(i); // Error: i is not defined (i is block-scoped)
// Example 7: Practical Usage for Variables that Need Reassignment
let greeting = "Hello";
greeting = "Hi"; // Allowed
console.log("Reassigned greeting:", greeting); // Output: Hi
// Example 8: Mutable References for Arrays and Objects with let
let fruits = ["apple", "banana"];
fruits.push("cherry"); // Allowed
console.log("Fruits array:", fruits); // Output: ["apple", "banana", "cherry"]
let car = { brand: "Toyota", model: "Corolla" };
car.model = "Camry"; // Allowed
console.log("Car object:", car); // Output: { brand: "Toyota", model: "Camry" }
Explanation of Code
- Block Scope: Variables declared with
let
are accessible only within the block where they are defined, just likeconst
. - Reassignment: Variables declared with
let
can be reassigned new values. - Mutable References: Objects and arrays declared with
let
can be modified. - No Initialization Required: Unlike
const
,let
does not require initialization at the time of declaration. - No Re-declaration in the Same Scope:
let
prevents re-declaration within the same scope, helping avoid accidental overwrites. - Block-Scoped Behavior in Loops:
let
is commonly used in loops because it ensures the loop variable is block-scoped. - Practical Usage:
let
is used for variables that need reassignment, providing flexibility.
The let
keyword is a powerful and flexible tool for declaring variables in JavaScript. Its block-scoped behavior, ability to allow reassignment, and prevention of redeclaration make it an essential feature for writing clean and error-free code. Whether you’re working on small scripts or large projects, understanding and utilizing let
effectively will enhance your coding practices.
By replacing var
with let
in most cases, you ensure better scoping and fewer accidental bugs in your JavaScript applications.
What is Function In JavaScript and how it Works ?
And the other thing is function on JavaScript. Function is like a heart of JavaScript, These is the most beautiful and best thing in JavaScript. Everything whatever happens inside the JavaScript, It happens because of the function.
You Can’t learn JavaScript without learning function, And not only function is important in JS. This also play an important role on React JS. Everything happen on a website like clicking a button, This all thing happen because of the function. And this is reason function play an important role in frontend development part.
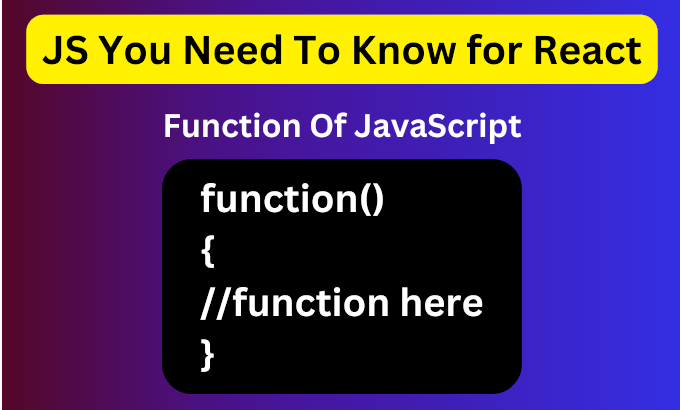
There are the Some Function Of JavaScript which is also in react JS.
- Arrow Function
- Higher order Function
Inside the function you should know about this two function. You should know how to write the syntax of all this function. Don’t worry i will explain all this two function in brief, After knowing all this function then your most of the learning react JS journey is completed.
How Arrow Function Work In JavaScript And React JS?
Arrow functions, introduced in ES6 (ECMAScript 2015), are a shorthand way of writing functions in JavaScript. They simplify function syntax and offer more predictable behavior when it comes to this
binding, which is especially helpful in React JS. Let’s explore arrow functions, how they work, and why they are so useful, with practical examples.
// Basic Arrow Function
const greet = (name) => `Hello, ${name}!`;
console.log(greet("Abhishek")); // Output: Hello, Abhishek!
// Arrow Function with Implicit Return
const multiply = (a, b) => a * b;
console.log(multiply(5, 4)); // Output: 20
// Arrow Function and `this` Binding
function RegularFunction() {
this.value = 10;
setTimeout(function () {
console.log("Regular Function:", this.value); // Undefined, `this` refers to the global object
}, 1000);
}
function ArrowFunction() {
this.value = 10;
setTimeout(() => {
console.log("Arrow Function:", this.value); // Output: 10, `this` inherits from surrounding scope
}, 1000);
}
new RegularFunction();
new ArrowFunction();
// Arrow Function in React JS - Functional Component
const Welcome = (props) => {
return <h1>Hello, {props.name}!</h1>;
};
// Example Usage
// <Welcome name="Abhishek" />;
// Arrow Function as Event Handler in React
class Counter extends React.Component {
state = { count: 0 };
// Arrow function for automatic `this` binding
increment = () => {
this.setState({ count: this.state.count + 1 });
};
render() {
return (
<div>
<p>Count: {this.state.count}</p>
<button onClick={this.increment}>Increment</button>
</div>
);
}
}
// Async/Await with Arrow Functions (Promises Example)
const fetchData = async (url) => {
try {
const response = await fetch(url);
const data = await response.json();
console.log("Fetched Data:", data);
} catch (error) {
console.error("Error fetching data:", error);
}
};
// Example Usage
// fetchData("https://api.example.com/data");
// Combining Arrays with Map (Arrow Functions in Array Methods)
const numbers = [1, 2, 3, 4, 5];
const doubledNumbers = numbers.map((num) => num * 2);
console.log(doubledNumbers); // Output: [2, 4, 6, 8, 10]
Explanation of the Code
- Basic Arrow Functions: Show the concise syntax and implicit return with the
greet
andmultiply
functions. - Arrow Function and
this
Binding: Demonstrates the difference between regular and arrow functions in handlingthis
. - React Functional Component: Example of a simple React functional component using an arrow function.
- Event Handler in Class Components: Highlights the use of arrow functions to avoid manual
this
binding in React class components. - Async/Await with Arrow Functions: Illustrates how arrow functions are used with modern features like
async/await
for fetching data. - Arrow Functions in Array Methods: Demonstrates the use of arrow functions in array methods like
map
.
This combined code showcases various use cases of arrow functions in both JavaScript and React JS, making it easier to understand their flexibility and power.
How Higher order Function Function Work In JavaScript And React JS?
Higher Order Functions (HOF) are functions that either take one or more functions as arguments or return a function as a result. They are one of the fundamental features of JavaScript and are widely used in React JS for handling various tasks, such as event handling, state management, and array manipulation
In this post, we’ll explain what higher order functions are, how they work, and how they are used with practical examples.
const numbers = [1, 2, 3, 4, 5];
// Using map and arrow function to double the numbers
const doubledNumbers = numbers.map((num) => num * 2);
console.log(doubledNumbers); // Output: [2, 4, 6, 8, 10]
// Using filter and arrow function to get even numbers
const evenNumbers = numbers.filter((num) => num % 2 === 0);
console.log(evenNumbers); // Output: [2, 4]
// Using reduce and arrow function to get the sum of numbers
const sum = numbers.reduce((accumulator, num) => accumulator + num, 0);
console.log(sum); // Output: 15
In this case, arrow functions are used as the arguments for map()
, filter()
, and reduce()
, making the code concise and readable.
Higher Order Functions are an essential part of JavaScript and React JS. They allow for cleaner, more reusable code by enabling functions to accept other functions as arguments or return them as values. In React, HOFs are commonly used for event handling, managing state, and rendering dynamic content.
By combining arrow functions with higher order functions, developers can create efficient and expressive code. Mastering these concepts will help you write cleaner, more maintainable JavaScript and React applications.
What is Array and Objects in JavaScript ?
Now after knowing the basic and function of JavaScript you have covered most of the part in the journey of learning the react JS. A lot of thing we will do while building an web app it is about array and objects. You can not avoid array and objects, while working on a react or any other JS library.
So you should know how to deals with array and objects, you have to know how array work and how to handle array. Don’t worry i will explain all of this in very detail, so you guys can understand quickly in simple language.
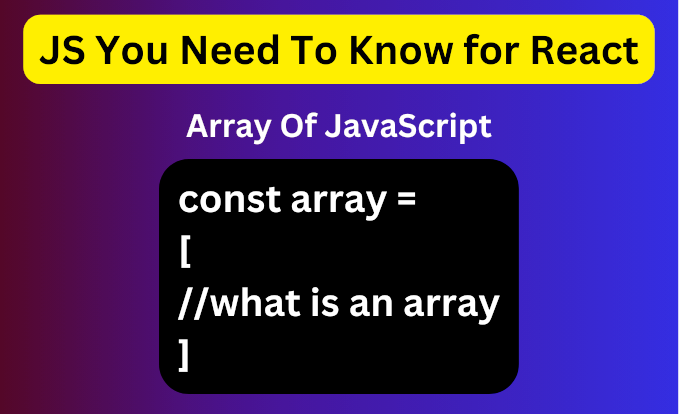
So In the array and object you have to learn all this things listed below.
- Array and Objects
- Array Destructuring
- Object Destructuring
- Rest Operator
- Spread Operator
This is all you need to learn in array and object, And learning this is an also an important thing before going towards the react js. Array and objects help you to manage large number of data while building a large web application.
What is Array and Objects in JavaScript ?
Arrays and objects are fundamental data structures in JavaScript that play a crucial role in React development. They help in managing, organizing, and displaying data dynamically on the user interface. This blog will provide an in-depth explanation of arrays and objects. Along with practical examples, focusing on how they are used in JavaScript and React JS.
// Array of objects representing a team
const team = [
{ name: 'Alice', age: 28, role: 'Developer' },
{ name: 'Bob', age: 34, role: 'Designer' },
{ name: 'Charlie', age: 29, role: 'Product Manager' }
];
// Add a new team member using the spread operator
const newMember = { name: 'Diana', age: 26, role: 'QA Engineer' };
const updatedTeam = [...team, newMember];
// Extract specific information from objects using destructuring
updatedTeam.forEach(({ name, role }) => {
console.log(`${name} works as a ${role}`);
});
// Filter out team members above 30 years old
const experiencedTeam = updatedTeam.filter(member => member.age > 30);
// Create a summary object with total team size and average age
const summary = updatedTeam.reduce(
(acc, member) => {
acc.totalAge += member.age;
acc.totalMembers += 1;
return acc;
},
{ totalAge: 0, totalMembers: 0 }
);
// Calculate the average age
summary.averageAge = summary.totalAge / summary.totalMembers;
console.log('Updated Team:', updatedTeam);
console.log('Experienced Team:', experiencedTeam);
console.log('Team Summary:', summary);
Output of the code
Alice works as a Developer
Bob works as a Designer
Charlie works as a Product Manager
Diana works as a QA Engineer
Updated Team: [
{ name: 'Alice', age: 28, role: 'Developer' },
{ name: 'Bob', age: 34, role: 'Designer' },
{ name: 'Charlie', age: 29, role: 'Product Manager' },
{ name: 'Diana', age: 26, role: 'QA Engineer' }
]
Experienced Team: [
{ name: 'Bob', age: 34, role: 'Designer' }
]
Team Summary: {
totalAge: 117,
totalMembers: 4,
averageAge: 29.25
}
This combined example demonstrates how arrays and objects work together to manage and manipulate data. It shows adding elements using the spread operator, extracting information with destructuring, filtering data, and summarizing it using reduce
. These techniques are crucial for handling real-world data in JavaScript and React JS applications.
Learn About Array Destructuring
Array destructuring allows us to unpack values from an array into distinct variables in a clean and concise way. It’s a powerful feature of JavaScript, especially useful for handling data effectively in modern applications.
Here is an example of Array Destructuring and how this work.
// Example 1: Basic Destructuring
const numbers = [10, 20, 30, 40, 50];
const [first, second, , fourth] = numbers; // Skipping the third value
console.log(`First: ${first}, Second: ${second}, Fourth: ${fourth}`);
// Example 2: Destructuring with Default Values
const colors = ['red', 'green'];
const [primary, secondary, tertiary = 'blue'] = colors; // Default value for missing elements
console.log(`Primary: ${primary}, Secondary: ${secondary}, Tertiary: ${tertiary}`);
// Example 3: Swapping Variables with Destructuring
let a = 1, b = 2;
[a, b] = [b, a];
console.log(`After Swapping: a = ${a}, b = ${b}`);
// Example 4: Nested Array Destructuring
const nestedArray = [1, [2, 3], 4];
const [one, [two, three], four] = nestedArray;
console.log(`One: ${one}, Two: ${two}, Three: ${three}, Four: ${four}`);
// Example 5: Rest Operator with Destructuring
const fruits = ['apple', 'banana', 'cherry', 'date'];
const [firstFruit, ...restFruits] = fruits;
console.log(`First Fruit: ${firstFruit}`);
console.log(`Rest of the Fruits: ${restFruits.join(', ')}`);
// Example 6: Destructuring Function Return Values
function getCoordinates() {
return [100, 200];
}
const [x, y] = getCoordinates();
console.log(`X: ${x}, Y: ${y}`);
Output of the Code
First: 10, Second: 20, Fourth: 40
Primary: red, Secondary: green, Tertiary: blue
After Swapping: a = 2, b = 1
One: 1, Two: 2, Three: 3, Four: 4
First Fruit: apple
Rest of the Fruits: banana, cherry, date
X: 100, Y: 200
Explanation Of the Code
- We unpack the first, second, and fourth elements from the
numbers
array. The third element is skipped using a comma. - The
colors
array has only two elements. While destructuring, we provide a default value'blue'
for the missing third element. - We swap the values of
a
andb
in a single line using destructuring. - We unpack values from a nested array structure.
two
andthree
are extracted from the inner array. - The rest operator (
...
) collects the remaining elements of the array into a new array calledrestFruits
. - The
getCoordinates
function returns an array of two values. We use destructuring to assign them tox
andy
.
Array destructuring is a concise and readable way to extract data from arrays. It simplifies working with arrays, especially when handling nested data, skipping elements, assigning default values, or swapping variables. This feature is widely used in modern JavaScript and frameworks like React to manage data efficiently.
How to do Object Destructuring in JavaScript
Object destructuring allows us to extract properties from objects and assign them to variables with a clean and readable syntax. It simplifies the process of accessing object properties, especially when dealing with nested structures or default values.
Let’s Understand with an Example of some line of code.
// Example 1: Basic Object Destructuring
const user = {
name: 'John Doe',
age: 30,
location: 'New York'
};
const { name, age, location } = user;
console.log(`Name: ${name}, Age: ${age}, Location: ${location}`);
// Example 2: Destructuring with Default Values
const product = {
title: 'Laptop',
price: 1000
};
const { title, price, stock = 50 } = product; // Default value for 'stock'
console.log(`Title: ${title}, Price: ${price}, Stock: ${stock}`);
// Example 3: Destructuring with Renaming Variables
const employee = {
empName: 'Alice',
empAge: 25
};
const { empName: employeeName, empAge: employeeAge } = employee;
console.log(`Employee Name: ${employeeName}, Employee Age: ${employeeAge}`);
// Example 4: Nested Object Destructuring
const company = {
name: 'TechCorp',
address: {
city: 'San Francisco',
zip: 94107
}
};
const { address: { city, zip } } = company;
console.log(`City: ${city}, ZIP Code: ${zip}`);
// Example 5: Destructuring with Rest Operator
const student = {
id: 101,
name: 'David',
grade: 'A',
age: 20
};
const { id, ...otherDetails } = student;
console.log(`Student ID: ${id}`);
console.log('Other Details:', otherDetails);
// Example 6: Destructuring Function Parameters
function displayBook({ title, author, year }) {
console.log(`Title: ${title}, Author: ${author}, Year: ${year}`);
}
const book = {
title: 'The Great Gatsby',
author: 'F. Scott Fitzgerald',
year: 1925
};
displayBook(book);
Output of the code
Name: John Doe, Age: 30, Location: New York
Title: Laptop, Price: 1000, Stock: 50
Employee Name: Alice, Employee Age: 25
City: San Francisco, ZIP Code: 94107
Student ID: 101
Other Details: { name: 'David', grade: 'A', age: 20 }
Title: The Great Gatsby, Author: F. Scott Fitzgerald, Year: 1925
Explanation of the code.
- Properties from the
user
object are directly extracted into variables. - The
product
object does not have astock
property, so we assign a default value of50
. - We rename the
empName
andempAge
properties toemployeeName
andemployeeAge
. - We access nested properties (
city
andzip
) from theaddress
object inside thecompany
object. - The rest operator collects the remaining properties of the
student
object into a new object calledotherDetails
. - We destructure the
book
object directly in the function parameters to access its properties easily.
Object destructuring in JavaScript is a powerful tool for extracting data from objects, simplifying code, and improving readability. It works seamlessly with default values, renaming variables, nested structures, and even function parameters. This feature is widely used in modern JavaScript and frameworks like React for managing and passing data effectively.
What is Rest Operator in javaScript
The rest operator (...
) is a feature in JavaScript that allows us to collect all remaining properties of an object or elements of an array into a new variable. It’s particularly useful for handling dynamic data and extracting parts of objects or arrays.
Let’s Understand with an code example.
// Example 1: Rest Operator in Object Destructuring
const user = {
name: 'John Doe',
age: 30,
location: 'New York',
profession: 'Developer'
};
const { name, ...otherDetails } = user;
console.log(`Name: ${name}`);
console.log('Other Details:', otherDetails);
// Example 2: Rest Operator in Array Destructuring
const numbers = [1, 2, 3, 4, 5];
const [first, second, ...restNumbers] = numbers;
console.log(`First Number: ${first}, Second Number: ${second}`);
console.log('Rest of the Numbers:', restNumbers);
// Example 3: Rest Operator in Function Parameters
function sum(...args) {
return args.reduce((acc, num) => acc + num, 0);
}
console.log('Sum:', sum(1, 2, 3, 4, 5));
// Example 4: Combining Arrays with Rest Operator
const fruits = ['Apple', 'Banana', 'Mango'];
const vegetables = ['Carrot', 'Potato', 'Tomato'];
const combined = [...fruits, ...vegetables];
console.log('Combined Array:', combined);
// Example 5: Nested Rest Operator in Destructuring
const person = {
id: 101,
name: 'Alice',
address: {
city: 'San Francisco',
zip: 94107,
country: 'USA'
},
role: 'Designer'
};
const { address: { city, ...restAddress }, ...restPerson } = person;
console.log(`City: ${city}`);
console.log('Rest Address:', restAddress);
console.log('Rest Person Details:', restPerson);
// Example 6: Filtering Object Properties with Rest Operator
const car = {
brand: 'Tesla',
model: 'Model 3',
year: 2021,
color: 'Red'
};
const { color, ...carDetails } = car;
console.log('Car Details without Color:', carDetails);
Output of the code.
Name: John Doe
Other Details: { age: 30, location: 'New York', profession: 'Developer' }
First Number: 1, Second Number: 2
Rest of the Numbers: [ 3, 4, 5 ]
Sum: 15
Combined Array: [ 'Apple', 'Banana', 'Mango', 'Carrot', 'Potato', 'Tomato' ]
City: San Francisco
Rest Address: { zip: 94107, country: 'USA' }
Rest Person Details: { id: 101, name: 'Alice', role: 'Designer' }
Car Details without Color: { brand: 'Tesla', model: 'Model 3', year: 2021 }
Explanation of the code.
- Extract the
name
property from theuser
object and group the remaining properties (age
,location
,profession
) intootherDetails
. - Extract the first two elements of the
numbers
array intofirst
andsecond
, and group the remaining elements intorestNumbers
. - The
sum
function uses the rest operator to collect all arguments passed into the function as an array (args
), making it easy to calculate their sum. - The rest operator (
...
) spreads the elements offruits
andvegetables
arrays into a new combined array. - Extract the
city
property from theaddress
object and group the rest (zip
,country
) intorestAddress
. Similarly, group the remaining properties ofperson
intorestPerson
. - Remove the
color
property from thecar
object and group the remaining properties intocarDetails
.
The rest operator is a powerful and versatile feature in JavaScript that simplifies working with objects and arrays. It helps in extracting specific properties, collecting remaining data, and creating dynamic functions. Its applications in destructuring and function arguments make it an essential tool for writing clean and concise code.
If Else Condition in JavaScript
Before you move forward on creating a project with React JS. But you don’t know about If Else statement then i think you are not good to go for react.
This is also an important factor while working on any we project or working with js library. You should know about how to write a condition in JavaScript using If Else statement. You should aware about how to deal with condition while working on any project.
If you don’t know how to write this type of condition, Then don’t worry i will explain you to handle and write this type of statement.
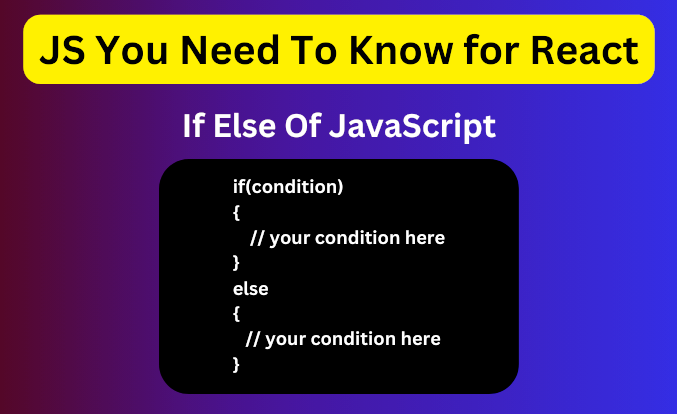
There are different type of condition type which you should know about them listed bellow.
- If Else
- Ternary Operator
- Using && and ||
- Optional Chaining
This is all a condition type statement and you should have a knowledge about them. This type condition can help you while creating any project with react or any other JavaScript library.
Map Filter and Reduce in JavaScript
The Next but important thing to know in JavaScript is Map filter and reduce function. This type of function can literally help you while dealing with an array. This are the function which is working with array to display on UI, filter any thing from array and many other thing.
And this is all the basics of JavaScript and you should know about it. All this function is given by the JavaScript and you can’t avoid it. While working on a react you have to use map function very fluently, It help you to display the array on UI.
Without doing the hard code you can just create an array and display to the Website interface using map function. But to do this you should have a knowledge about all this function, Not only the map function there are many function which help you to deal with an array.
There are the different type of function which help you handle or deal with the array.
- Map()
- Filter()
- Reduce()
- sort()
So this are the some JavaScript function which you should aware about for getting start with React JS. Trust me this type of function is going to help in you react learning journey. And this will also help you while creating an any project with your react or any JS library.
Don’t worry if you don’t know how to write this type of function i will guide you how to write this type of function. And how this will help in your react journey or creating an any project with any JS library.
What is Event Listeners in JavaScript for React JS ?
The most important thing you should know how to call event. If you know about how to write an function but you don’t know how to call a function. Then how the function will work, Event listeners is important because you can call the function using them.
Like you are in website and you click on random button and one popup appears. Or you are in the ecommerce website and you click Add to cart button, then the product is added to cart. But how all this things are possible.
This all things are possible because of the event listeners. The simple meaning of an event listeners it calls the function when some event is done. Like in Ecommerce website clicking an Add to cart button is an event and on that event the add to cart function is called. This is working process of an event Listeners.
Now i think you have a clear understanding of event listeners. There are the different type of event listeners, And each event listeners has different role. I have listed some of them, let’s know more about it.
- onClick
- onBlur
- onChange
- onFocus
- setTimeout()
- setInterval()
This are the some the basic event listeners. There are many more listeners accept them, But this are the basic one. You should have a knowledge about them, And all this listeners are going to help in your any JavaScript project like React JS.
If you guys don’t know about all this event listeners and don’t know how to write them in the any projects. I am here to explain you how to write an event listeners and how to call a function using them. I very detail and simple language i will try to explain so you can understand easily. Because this are most important things to know for creating any app.
What is Promises and Callbacks in JavaScript For React ?
This is also an important thing you should aware about it. Without promises you cannot work with any rest API. Or you are not able to call API, This help you to understand API and how to call them to implement to the project.
In any API related JS project the knowledge promises is necessary and you should have a knowledge about them. Without knowing promises you can not go to create any real time react project. Because it will hard to handle any API, And if this is the API based project and you don’t have a knowledge about promises. Then it’s going to be a nightmare for you.
If you guys thinking what does promises mean?. Let me answer your simple question, The term promises in JavaScript is mean by. Whenever you call an API then you have to pass the promises to API.
Promises is not necessary for every project. But if you working on project which needs an API call then the knowing about promises is become necessary for you. Because without promise you are not able to call an API.
There are some promises which help you handle the API listed bellow .
- Callbacks
- Callback Hell
- Promises
- Promise APIs
So this are the some promises which you should know as a React developer. This help you to work with API very smoothly.
Async/Await in JavaScript
This is an one of the type of Promises call, This is like a sugarcoating in promises. And this is one of the easiest way to call API or work with an APIs. Async await is used by modern developers because this is very easy to use. You don’t have to write complicated code to fetch API
You just need to write few line of code to work with an API or fetch an rest APIs. But you guys don’t know how to write an syntax of async await. Don’t worry i will guide you how to use async await and where to use and why to use. Everything you need to know about this i will tech you.
Let’s understand async/await with an example of code.
// Example 1: Basic Async/Await Function
async function fetchData() {
try {
const response = await fetch('https://jsonplaceholder.typicode.com/posts/1');
const data = await response.json();
console.log('Fetched Data:', data);
} catch (error) {
console.error('Error fetching data:', error);
}
}
fetchData();
// Example 2: Async/Await with Multiple Promises
async function fetchMultipleData() {
try {
const [post, user] = await Promise.all([
fetch('https://jsonplaceholder.typicode.com/posts/1').then(res => res.json()),
fetch('https://jsonplaceholder.typicode.com/users/1').then(res => res.json())
]);
console.log('Post Data:', post);
console.log('User Data:', user);
} catch (error) {
console.error('Error fetching multiple data:', error);
}
}
fetchMultipleData();
// Example 3: Async Function Returning a Value
async function addNumbers(a, b) {
return a + b;
}
addNumbers(5, 7).then(result => console.log('Sum:', result));
// Example 4: Using Async/Await in a Loop
async function fetchPosts(ids) {
for (const id of ids) {
try {
const response = await fetch(`https://jsonplaceholder.typicode.com/posts/${id}`);
const post = await response.json();
console.log(`Post ${id}:`, post);
} catch (error) {
console.error(`Error fetching post ${id}:`, error);
}
}
}
fetchPosts([1, 2, 3]);
// Example 5: Simulating Delays with Async/Await
function delay(ms) {
return new Promise(resolve => setTimeout(resolve, ms));
}
async function delayedMessage() {
console.log('Wait for it...');
await delay(2000); // 2-second delay
console.log('Here it is!');
}
delayedMessage();
// Example 6: Nested Async Functions
async function outerFunction() {
async function innerFunction() {
return 'Inner Function Result';
}
const result = await innerFunction();
console.log('Outer Function Result:', result);
}
outerFunction();
output of code.
Fetched Data: { userId: 1, id: 1, title: '...', body: '...' }
Post Data: { userId: 1, id: 1, title: '...', body: '...' }
User Data: { id: 1, name: '...', username: '...', ... }
Sum: 12
Post 1: { userId: 1, id: 1, title: '...', body: '...' }
Post 2: { userId: 1, id: 2, title: '...', body: '...' }
Post 3: { userId: 1, id: 3, title: '...', body: '...' }
Wait for it...
Here it is!
Outer Function Result: Inner Function Result
Explanation of code.
- The
fetchData
function fetches data from an API, waits for the response, and logs the result. Thetry/catch
block handles any errors. Promise.all
is used to wait for multiple promises to resolve. Data from two different endpoints is fetched simultaneously.- The
addNumbers
function demonstrates that an async function always returns a promise, even when returning a direct value. - The
fetchPosts
function iterates over an array of IDs, fetches data for each, and logs the result. This ensures sequential execution. - The
delay
function creates a promise that resolves after a specified time. ThedelayedMessage
function demonstrates how to use it. - An
outerFunction
calls aninnerFunction
usingawait
, demonstrating how async functions can be nested.
The async/await
syntax makes handling asynchronous code much more readable and maintainable compared to traditional promise chaining. By leveraging async/await
, you can write cleaner and more understandable asynchronous logic for tasks like API calls, delays, and sequential operations.
Try and Catch in JavaScript
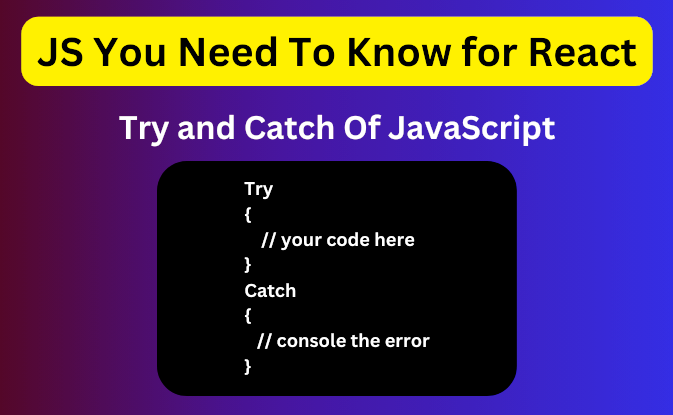
So what does mean by try and catch?, By the word try catch mean we have to try something and we want to catch something. But what is mean in term of JS or in React JS. I will give the answer of your all question, And i will also show how this work and how to write this type of statement.
The Try Catch statement used to handle error in code. But how, when we write some line of code then we have to put them inside try catch statement. So if there will be an error then we can console the error. It become easy to find and solve the error.
There will be many bugs or error when you will work on big project like ecommerce and many more. Then you should know about it how to write try and catch statement. And how to solve the error using this. This is very helpful thing which is used to solve or find error or bugs in code.
Let’s take an Example using code.
try {
// Code that may throw an error
} catch (error) {
// Code to handle the error
} finally {
// Optional block for cleanup (executed regardless of success or failure)
}
The try...catch
block is essential for writing robust JavaScript code. It helps in handling runtime errors gracefully, avoiding program crashes. The optional finally
block ensures cleanup operations, making it a powerful tool for error handling and resource management in any JavaScript application.
Conclusion of How Much JavaScript You Should Know For React
So the final answer of your question is you should have a basic knowledge of JavaScript before getting started with React JS. In this Post I have covered all the JS concept that you must know to getting started with react JS , Or to start you react web development journey.
Mastering the foundational concepts of JavaScript is crucial before diving into React JS. In this blog post, I have outlined the key topics in JavaScript that will smoothen your journey into React development. From understanding variables, functions, and arrays to exploring advanced concepts like promises, async/await, and event listeners, these building blocks form the backbone of your web development skills.
With a solid grasp of these topics, you’ll be well-equipped to handle the challenges of React JS, create dynamic web applications, and tackle real-world projects with confidence. Remember, every concept covered here is a stepping stone toward mastering React and building robust, scalable applications.
If you’re ready to embark on your React learning journey, ensure you revisit these JavaScript fundamentals. With consistent practice and dedication, the path to becoming a proficient React developer will be clear and achievable. Happy coding!